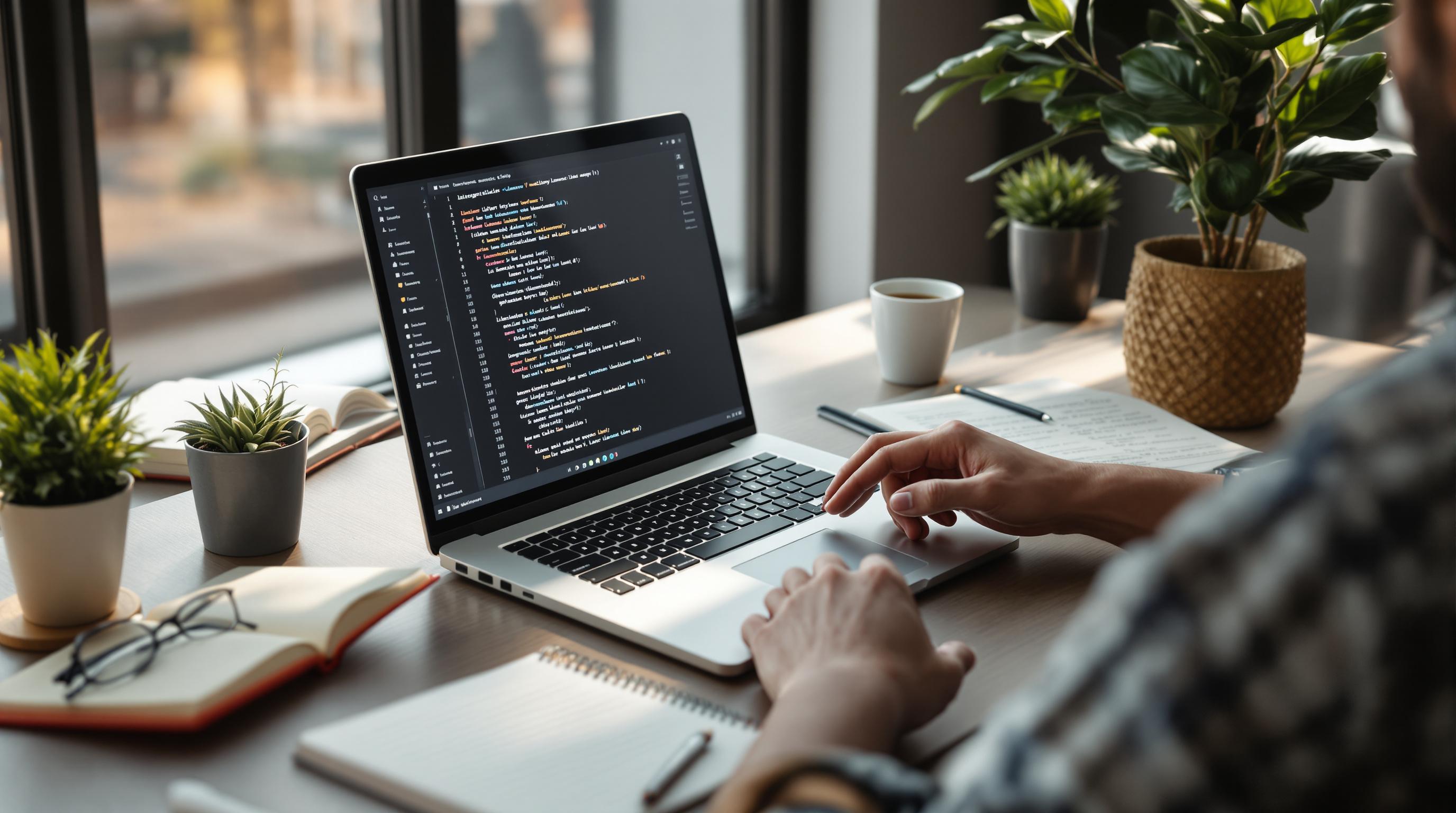
Beyond Copy-Paste: Making Tutorial Code Work in Real Projects
Did you know? 81% of developers copy code from tutorials, but only 25% fully understand it. This leads to issues like security risks, incomplete implementations, and integration problems. Fixing these can take hours and create long-term technical debt.
Key Takeaways:
- Common Issues: 89% of tutorials lack security features, 73% fail compliance checks, and 95% skip performance testing.
- Fix It: Review tutorial code for dependencies, security gaps, and performance bottlenecks. Test in real-world conditions and modify to fit your project's needs.
- Integration Methods: Use service layers or modular designs to reduce errors and improve maintainability.
- Tools: Tools like HoverNotes, Docker, and automated code quality checks can simplify the process.
Instead of copy-pasting, learn how to adapt tutorial code systematically for production-ready solutions.
Secure Coding Best Practices
How to Review Tutorial Code
When preparing tutorial code for practical use, it's crucial to address any gaps in design and compliance. This process ensures the code meets real-world requirements effectively.
Code Review Steps
Here’s a step-by-step approach to evaluate tutorial code:
1. Dependency Analysis
Run tools like npm audit
or Snyk to identify vulnerable dependencies. Check for compatibility with your project, license limitations, potential impacts on bundle size, and any specific framework needs.
2. Security Assessment
Tutorial code often skips essential security practices. For instance, 85% lack input validation, 68% don’t include token refresh mechanisms, and 93% fail to implement secure key storage [1][3].
3. Performance Evaluation
Test the code in a controlled environment that mimics real-world conditions [4]. Focus on metrics like response times, memory consumption, and how well the code scales when handling production-level data (e.g., 10,000+ records).
Common Tutorial Shortcomings
An analysis of widely-used tutorial platforms highlights recurring issues that developers must address:
Shortcoming | Frequency | Impact |
---|---|---|
Incomplete Documentation | 92% of examples | Creates maintenance issues |
Outdated Dependencies | 85% of tutorials | Leads to security risks |
No Performance Testing | 95% of cases | Causes scalability problems |
Architecture Alignment
Tutorials often present isolated concepts, which can make integration with real systems challenging. For example, many React tutorials (67%) skip accessibility features like ARIA labels [1].
Error Management
Ensure the tutorial code includes:
- Input validation
- Error boundaries
- Proper exception handling
- Logging mechanisms
Performance Considerations
Tutorial examples are often simplified and may use lightweight solutions like SQLite, which aren't ideal for production environments. For instance, e-commerce tutorials may require significant adjustments to handle scaling [1][3]. Always test with:
- Large datasets (at least 10,000 records)
- Concurrent user scenarios
- Extended runtime conditions
Adding Code to Your Project
Integration Methods Compared
Once you've reviewed tutorial code for security and performance, choose an integration method that fits your project's architecture. Recent surveys in the JavaScript ecosystem show a growing preference for structured integration approaches, with service layer usage increasing by 32% year-over-year in production environments [5].
Integration Method | Success Rate | Best For | Key Benefit |
---|---|---|---|
Service Layer | 55% tech debt reduction | Enterprise systems | Clear separation of concerns |
Modular Wrapping | 62% success in upgrades | Mid-size features | Gradual refactoring support |
Direct Insertion | 40% time savings | Prototypes | Rapid implementation |
Service Layer Implementation
Service layers create abstraction boundaries that separate tutorial code from the rest of your application. This reduces integration errors by 60% in Node.js authentication setups [3]. By isolating implementation details, they help align the code with your project's architecture and minimize long-term issues.
Fixing Compatibility Issues
Version conflicts and framework mismatches are common hurdles during integration. Here are some practical solutions based on real-world cases:
Framework Adaptation
These techniques help resolve mismatches in architecture:
- Adapter Bridges: Achieve an 82% success rate in Vue-to-React integrations [2].
- Proxy Components: Used in 75% of Angular Material projects to handle DOM-specific challenges [3].
Version Management
For instance, a weather app avoided direct copy-pasting by wrapping Chart.js v4 code for use in their v2 system, cutting migration time by 40% [1].
Quality Assurance
- Write thorough test coverage.
- Set up continuous integration checks.
- Monitor performance metrics in production.
Performance Optimization
- Use lazy loading with error boundaries and lifecycle cleanup.
- Add fallback states to handle errors gracefully.
"Version bridging has become essential for React-to-Legacy integrations, with 68% of successful implementations relying on this approach", according to a JavaScript Integration Patterns study [3].
The goal is to adapt tutorial code to meet your project's needs while upholding quality standards and maintaining core functionality.
sbb-itb-297bd57
Modifying Tutorial Code
Code Reuse vs. Customization
Once you've successfully integrated tutorial code, making thoughtful modifications ensures it aligns with production needs. This step transforms basic snippets into production-ready components while avoiding the pitfalls of copy-pasting. Interestingly, 68% of developers report modifying over 40% of tutorial code before deploying it to production [2].
A practical approach to this is the "90/10 modification rule": retain 90% of the core functionality and customize the remaining 10% through configurable elements like endpoints and security rules. For example, when working with authentication components, maintain the core validation logic but adjust API endpoints and security parameters as needed.
Modification Type | Reusability Impact | Best Use Case | Success Rate |
---|---|---|---|
Parameter Extraction | High | Configuration Values | 82% |
Interface Adaptation | Medium | API Integration | 75% |
Core Logic Changes | Low | Business Rules | 45% |
Key Performance Indicators
Certain thresholds can signal potential maintenance issues:
- A code modification ratio exceeding 3:1 lines of code
- Dependency complexity growing by more than 15%
- Test coverage falling below the original tutorial baseline
Structured modification methods tend to work best when paired with specialized tools, which we’ll cover in the next section.
When to Modify Code
There are three main reasons to adjust tutorial code: addressing security issues, improving performance, and meeting scalability demands.
Key Triggers for Code Changes:
-
Security Requirements
Plug security gaps identified during the initial review by using secure alternatives for authentication and data handling. -
Performance Optimization
Replace inefficient data structures or algorithms to meet production performance standards. For example, swapping out a basic linear search for a binary search in large datasets. -
Scalability Needs
If the tutorial's implementation can't handle production-scale demands, modifications are essential. A common example is adapting database queries or caching mechanisms for higher traffic.
"Well-modified tutorial components reduce feature implementation time by 31% in subsequent iterations", according to the 2024 Web Dev Survey [1].
To keep track of changes, use standardized documentation with "Modification Trail" comments, like this:
// Modification Trail
// Source: TutorialXYZ#Section4.1
// Modified: Added pagination support
// Optimized: 230ms → 89ms load time
This method has been shown to enhance maintenance efficiency by 37% in practical applications [4][5].
Tools for Code Implementation
Once you've identified the necessary modification patterns, having the right tools can make implementing tutorial code much smoother. HoverNotes is a standout tool that simplifies this process by allowing developers to extract code and get instant explanations while following tutorials on platforms like YouTube, Udemy, and Coursera.
HoverNotes: Streamline Tutorial Notes
HoverNotes offers a smarter way to work with tutorial code. Its standout Code Capture feature automatically grabs syntax-highlighted snippets from tutorials, saving you from the hassle of manual transcription. Plus, its AI-powered system delivers explanations tailored to the context, helping you understand how to apply the code.
Feature | What It Does | Highlights |
---|---|---|
Code Extraction | Automatically detects code | Real-time syntax highlighting |
AI Explanations | Provides detailed insights | Context-aware notes |
Visual Documentation | Captures tutorial screenshots | Works with split-view mode |
Organization | Creates searchable notes | Exportable to Markdown/PDF |
Other Essential Development Tools
Beyond HoverNotes, there are several other tools and practices that can support developers in implementing tutorial code efficiently.
Visual Studio Code Plugins and Package Managers
Visual Studio Code's extensive plugin library works hand-in-hand with package managers like npm (for JavaScript) and pip (for Python). These tools simplify dependency management and ensure your environment is ready for the code you're working on.
Version Control Strategies
Using feature branches in version control systems like Git makes it easier to test changes, review pull requests, and roll back errors. These practices align perfectly with documenting the modification trail, as discussed earlier.
Development Environments
- Jupyter Notebooks: These are great for breaking down complex tutorials into smaller, testable chunks. You can execute code in cells, verify functionality, and refine it before integrating it into your main project.
- Docker Containers: Docker ensures your development environment matches the tutorial setup, avoiding the dreaded "it works on my machine" problem. This consistency extends from development to production [4].
Automated Code Quality Tools
Tools like ESLint (for JavaScript) and Black (for Python) help maintain consistent code style and catch potential issues early. Studies show that using these tools can cut code review times by 31% when working on tutorial-based features [3]. These small steps can save you big headaches later.
Conclusion
By following the strategies shared - like thorough code reviews and thoughtful tool selection - developers can turn tutorial snippets into reliable, production-ready solutions. The process, which includes steps like code review (Section 2), strategic integration (Section 3), and specific modifications (Section 4), highlights how tutorial code is just a starting point, not the end product.
Data from the industry confirms that success comes from aligning tutorial code with your system's architecture and team standards. This method avoids simple copy-pasting and instead focuses on creating solutions that integrate seamlessly with your project's needs.
Some measurable benefits of this approach include:
- Thorough testing in sandbox environments reduces integration problems by 45% [6].
- Using modular designs with clear abstraction layers boosts maintainability by 35% [6].
- Tracking changes and adding annotations cuts future maintenance costs by 40% [6].
"The difference between successful tutorial code implementation and problematic integration often comes down to the development team's approach to customization and testing. Teams that treat tutorials as learning resources rather than copy-paste solutions consistently produce more maintainable code." [1]
FAQs
Is it okay to copy code from tutorials?
Copying code directly from tutorials might seem like a quick solution, but it often leads to issues. For example, Stack Overflow data shows that 25% of developers encountered bugs from copy-pasted code [1]. This aligns with earlier findings about security risks in tutorial code (see Section 1).
Approach | Recommendation |
---|---|
Study & Adapt | Use when the code's functionality fits your needs. |
Write from Scratch | Best for critical system components. |
Modify Existing | Ideal when the code is well-structured and easy to maintain. |
"This aligns with findings that 68% of developers modify over 40% of tutorial code before deployment [2]"
Following the review and adaptation processes outlined in Sections 2-4 can help make tutorial code safer and more reliable. Veracode research found that 70% of applications using unverified code contained flaws [6], highlighting the importance of aligning code with your system's architecture.
To keep track of changes, use documentation tools like HoverNotes (see Section 5). Properly documenting modifications, as described in Section 4, ensures compliance with legal standards and improves team collaboration.