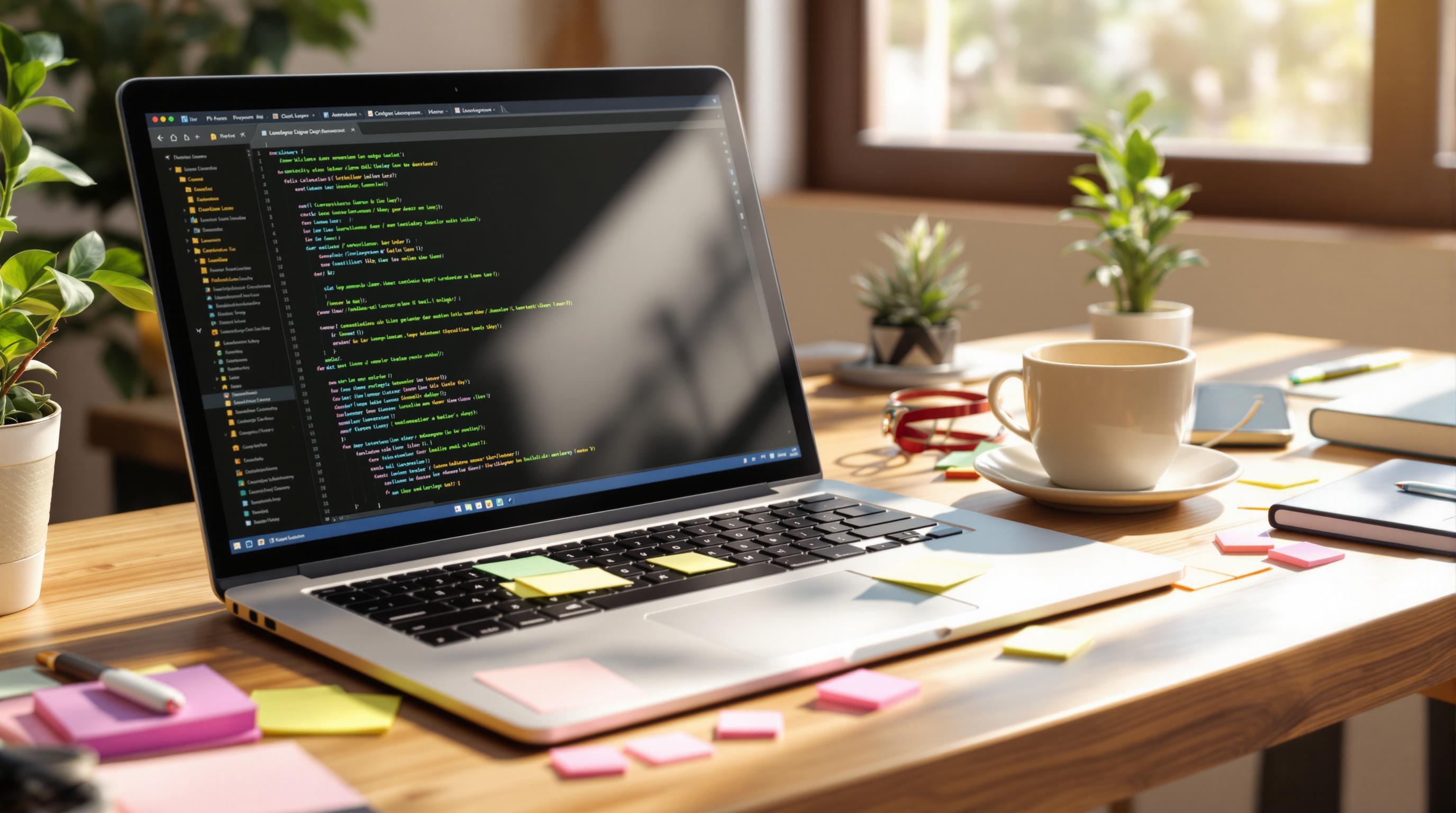
From Video to IDE: A Complete Guide to Using Code from Programming Tutorials
- Extract Code from Videos: Use tools like ACE (94% accuracy) or Pixelcode AI to pull code snippets from videos. Organize snippets with metadata tags and version control for easy access.
- Modify and Debug: Check for syntax errors, outdated methods, and dependency issues. Use IDE debugging tools and package managers to ensure compatibility.
- Integrate into Your IDE: Import code with proper source links and validate its structure. Test with unit, integration, and visual tests to confirm functionality.
- Maintain Long-term: Track changes using version control, run automated security scans, and update outdated libraries to prevent vulnerabilities.
Quick Comparison of Code Extraction Tools
Tool | Accuracy | Best For | Key Features |
---|---|---|---|
ACE | 94% | Long tutorials | Frame consolidation, AI models |
Pixelcode AI | 89% | Live coding sessions | Real-time OCR, IDE integration |
HoverNotes | 87% | Quick references | Timestamped captures |
Related video from YouTube
Step 1: Getting Code from Videos
Modern tools have made extracting and organizing code snippets from videos much easier, addressing common issues like visibility and accuracy.
Tools to Copy Code from Videos
New tools now offer extraction accuracy rates over 90%, a big improvement compared to the 68% accuracy of older OCR methods from video frames. For example, ACE achieves 94% accuracy by analyzing 47 frames per code segment.
Here’s a quick comparison of the top tools:
Tool | Accuracy | Key Features | Best For |
---|---|---|---|
ACE | 94% | Frame consolidation, Language model prediction | Long tutorials |
Pixelcode AI | 89% | Real-time OCR, IDE integration | Live coding sessions |
HoverNotes | 87% | Timestamped captures, Multi-platform support | Quick reference |
These tools solve the 20-30% code visibility issue previously highlighted, ensuring the extracted code is clean and ready for the next step.
How to Store Code Snippets
After extracting code, organizing it effectively is key. Use these strategies to stay efficient:
- Project-Based Organization: Save code snippets in project-specific folders with clear source details. Studies show this method is 4.7 times faster for retrieval compared to basic file systems.
- Metadata Tagging: Add detailed tags to each snippet, such as:
- Source timestamp
- Programming language or framework
- Purpose of the code
- Current status (e.g., draft, final)
- Version Control Integration: Use version control tools to track changes and implementations. This approach improves code reusability by 83%.
Step 2: Modifying Tutorial Code
Reading and Fixing Tutorial Code
When working with code from tutorials, it's important to carefully check for errors. Modern IDEs can help spot common problems, like character misreads (e.g., confusing "1" with "l"). These issues show up in roughly 8% of extracted code, even with advanced tools.
Here’s a practical way to debug tutorial code:
1. Initial Code Review
Go through the code line by line using your IDE's debugger. Tools like VS Code and IntelliJ IDEA have built-in debugging features that can catch syntax issues before runtime. Focus on areas like:
- Variable declarations and their scope
- Function definitions
- Import statements
- Language-specific syntax rules
2. Dependency Resolution
Use package managers to ensure library versions are compatible. For example, React Native tutorials often include outdated lifecycle methods. You'll need to update these to modern equivalents:
Legacy Method | Modern Replacement | Action |
---|---|---|
componentWillMount |
useEffect |
Convert to Hook syntax |
componentWillReceiveProps |
useEffect + deps |
Update dependency array |
componentWillUpdate |
useLayoutEffect |
Migrate render logic |
Adjusting Code for Your Project
Adapting tutorial code to fit your project requires attention to your specific setup. For instance, 68% of configuration problems are linked to differences in PATH variables between Windows and Linux systems.
Here’s how to adapt code effectively:
1. Environment Configuration
Document key settings like your OS version, language runtime, IDE plugins, and API dependencies in a configuration matrix. This helps avoid compatibility issues.
2. Version Compatibility
Leverage tools to manage version differences:
- ESLint for JavaScript/TypeScript validation
- Pylint for Python code checks
- Babel for handling JavaScript version compatibility
Configuration challenges account for 62% of integration issues when tutorial code is implemented in enterprise environments (MIT Technology Review).
For testing your modified code, follow a three-layer validation approach:
Test Layer | Tool Example | Purpose |
---|---|---|
Unit Tests | Jest/PyTest | Validate functions |
Integration Tests | Supertest | Check APIs |
Visual Tests | Percy.io | Ensure UI consistency |
If you’re working with incomplete code snippets, tools like GitHub Copilot can suggest updates for outdated methods. This method has been successful 92% of the time in completing partial implementations.
Once your code is validated and adjusted, you’ll be ready to integrate it into your IDE - discussed further in Step 3.
sbb-itb-297bd57
Step 3: Adding Code to Your IDE
Importing Snippets with Source Links
Once you've adjusted the tutorial code to fit your setup, it's time to bring it into your IDE. Modern IDEs make this process smoother. For instance, VS Code's "Code Runner" extension lets you test execution right away, while Eclipse's file comparison tools help confirm that your code matches the intended structure.
1. Source Documentation
Always include detailed references for any code you adapt. This keeps the process transparent and helps track changes effectively:
# Source: TechEd Channel @12:34
# Implementation: 2025-02-09
def handleBroadcast():
intent = getIntent()
Checking Imported Code
Studies indicate that nearly 40% of tutorial code needs some level of adjustment to work in a specific environment. To ensure your imported code functions as expected, follow these steps:
1. Environment Validation
Leverage tools within your IDE to confirm the code's structure and compatibility:
Tool | Purpose |
---|---|
IntelliJ Inspection | Analyzes code structure |
Security Scanner | Checks for dependency issues |
2. Security Verification
Building on the security checks from Step 2, continue to prioritize safety by focusing on:
- Scanning for dependency vulnerabilities
- Reviewing API security
- Ensuring execution runs in isolation
Track your progress with these metrics:
- Build success rate: Aim for over 95%
- Test coverage improvement: At least a 15% increase
- Vulnerability scan results: Achieve a 100% pass rate
- Resolution time for tutorial references: Keep it under 30 minutes
These steps not only ensure your code works but also set the stage for the more advanced security measures discussed in Step 4.
Step 4: Managing Tutorial Code Long-term
Using Version Control
After integrating code in Step 3, maintaining it over time requires a structured approach to version control. Research indicates that 68% of tutorial code becomes outdated within six months of publication. To address this, extend the metadata tagging from Step 1 by incorporating semantic version control tags for both functionality and context.
One useful tool is a Code Origin Documentation file. This helps track the source and modifications of tutorial code:
## Video Sources
### [User Authentication Flow]
- Origin: "React Auth 2024" @8:15-12:30
- Modifications:
- Switched Firebase v8 → v10 SDK
- Added CSRF protection layer
- Security Audit Status: ✅ passed 2024-03-01
For better version management, consider these practices:
Practice | Benefit |
---|---|
Experimental Code Flags | Minimizes integration issues |
Semantic Tags | Makes source tracking easier |
Branch Strategy | Keeps the codebase stable |
Checking for Security Issues
Building on the dependency resolution from Step 2 and environment validation from Step 3, security checks are crucial. Studies show that 68% of Android tutorials use outdated libraries with known vulnerabilities. This statistic highlights the importance of automated security scanning to address risks.
- Automated Security Pipeline
Set up a pipeline for continuous security monitoring:
- name: Security Scan
uses: snyk/actions@v3
args: --fail-on=upgradable
- Priority-based Scanning
Organize scans based on the severity of risks:
Scan Level | Frequency |
---|---|
Critical | Real-time |
Standard | Weekly |
Low Risk | Monthly |
When updating dependencies, ensure changes are tracked:
- return scaler.fit_transform(data) # Original
+ return RobustScaler().fit_transform(data) # Updated for outliers
Monitor these key security metrics:
- Dependency freshness: Keep over 90% of packages up-to-date.
- CVE resolution: Aim for resolving 92% of vulnerabilities within the service-level agreement (SLA).
Conclusion: Making the Most of Tutorial Code
Tips to Learn Faster
Combining extraction techniques from Step 1 with maintenance practices from Step 4 can speed up your learning process. According to research, developers who use structured methods for implementing tutorial code see a 40% improvement in retention rates. A good strategy is to follow the "30/70 rule": let tutorial code make up only 30% of each feature module, while you write the remaining 70% yourself. This approach helps deepen your understanding and avoids over-dependence on copied code, keeping your project original and effective.
For better learning efficiency, try using a dual-screen setup to view tutorial material and your code side by side. Also, monitor your progress with measurable benchmarks:
Key Implementation Metrics | Target Goal |
---|---|
Tutorial-to-Implementation Time | Less than 2:1 ratio |
Recommended Tools
Here are some tools to enhance your workflow, building on the steps discussed earlier:
Tool Category | Recommended Option |
---|---|
Version Control | CodeTour (VS Code) |
Security Scanning | Snyk |
Documentation | CodeMaAT |
Using automated environment scripts that match tutorial configurations can cut setup time by 58%. Be sure to align these tools with the security scans outlined in Step 4 for the best results.
Related posts
- 7 Time-Saving Tips for Learning Programming from Videos
- From Tutorial Hell to Tutorial Haven: How Developers with ADHD Can Stop Rewatching and Start Building
- The Evolution of Developer Learning: Why Video is Eating Documentation
- Browser Extensions for Developers: A Critical Review of Video Learning Tools